D.1 Objects as a programming concept (Notes)
D.1 Objects as a programming concept (6 hours)
Syllabus D.1
Assessment statement | Obj | Teacher’s notes | |
D.1.1 | Outline the general nature of an object. | 2 | An object as an abstract entity and its components—data, and actions. Familiar examples from different domains might be people, cars, fractions, dates, and music tracks. |
D.1.2 | Distinguish between an object (definition, template or class) and instantiation. | 2 | Students must understand the difference in terms of code definitions, memory use, and the potential creation of multiple instantiated objects. |
D.1.3 | Construct unified modelling language (UML) diagrams to represent object designs. | 3 | LINK Connecting computational thinking and program design. |
D.1.4 | Interpret UML diagrams. | 3 | LINK Connecting computational thinking and program design. |
D.1.5 | Describe the process of decomposition into several related objects. | 2 | A simple example with 3–5 objects is suggested. Examples related to D.1.1 could be employers, traffic simulation models, calculators, calendars, and media collections. LINK Thinking abstractly. AIM 4 Applying thinking skills critically to decompose scenarios. |
D.1.6 | Describe the relationships between objects for a given problem. | 2 | The relationships that should be known are dependency (“uses”), aggregation (“has a”), and inheritance (“is a”). LINK Thinking abstractly. AIM 4 Applying thinking skills critically to decompose scenarios. |
D.1.7 | Outline the need to reduce dependencies between objects in a given problem. | 2 | Students should understand that dependencies increase maintenance overheads. |
D.1.8 | Construct related objects for a given problem. | 3 | In examinations, problems will require the students to construct definitions for no more than three objects and to explain their relationships to each other and any additional classes defined by the examiners. LINK Connecting computational thinking and program design. AIM 4 Applying thinking and algorithmic skills to resolve problems. |
D.1.9 | Explain the need for different data types to represent data items. | 3 | The data types will be restricted to integer, real, string, and Boolean. |
D.1.10 | Describe how data items can be passed to and from actions as parameters. | 2 | Parameters will be restricted to the pass-by-value of one of the four types in D.1.6. Actions may return at most one data item. |
D.1.1 Outline the general nature of an object.
Object
D.1.2 Distinguish between an object (definition, template or class) and instantiation.
Class
Instantiation
We can make lots of objects from the same class. Each object requires some memory to store its details. Each object has its own copy of its VARIABLES and its copy of the class method. But, if the methods or variables are static, then there is only one copy of the method or variable, and all the objects share the same copy.
Car car1 = new Car(); // Instantiating a Car object
Car car2 = new Car(); // Instantiating another Car object
Difference between Objects and Instantiation and a Class
An object is a specific instance of a class that has its unique state and behavior. It represents a runtime entity in memory. On the other hand, instantiation is the process of creating an object from a class.
When we say that a class is an abstract entity, it means that the class serves as a general concept or blueprint rather than a specific instance. It defines the common structure, behavior, and attributes that objects of that class will possess, but it cannot be directly instantiated. Abstract classes are used as a foundation for creating subclasses, which can be instantiated.
A class does not occupy memory space on its own. It is only when objects are instantiated from the class that memory is allocated to store the state and behavior of those objects. Each object created from a class has its own memory allocation, while the class itself remains abstract and does not consume memory.
D.1.3 Construct unified modelling language (UML) diagrams to represent object designs.
UML Diagrams
The purpose of a UML diagram is to facilitate communication between stakeholders, software developers, and designers by presenting a visual representation of the system's architecture. UML diagrams can represent different aspects of a system, such as class diagrams, use case diagrams, sequence diagrams, and more.
Example:
Train |
- mEngines: Engine[] - mWagons: Wagons[] - mEngineCount: int - mWagonCount: int - mTrainNumber: int - mWeight: double |
+ addEngine( Engine newEngine ): void + removeEngine(): Engine + addWagon( Wagon newWagon ): void + removeWagon(): Wagon + getWeight(): double |
Tutorial Links:


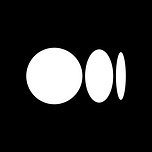

D.1.4 Interpret UML diagrams.
Example:
D.1.5 Describe the process of decomposition into several related objects.
Decomposition
Advantage of Decomposition
The advantage of decomposition is that it allows for better organization, modularity, and maintainability of code. By breaking down a system into smaller objects, each object can focus on a specific task or responsibility, making the code easier to understand, test, and modify. It promotes code reusability and facilitates collaboration among developers working on different components.
D.1.6 Describe the relationships between objects for a given problem.
- Association: Association represents a relationship between two or more objects where they are connected or interact with each other. It can be a one-to-one, one-to-many, or many-to-many relationship. For example, a "Teacher" object can be associated with multiple "Student" objects.
- Dependency: Dependency is a relationship between objects where one object depends on another object for some functionality or resources. It indicates that a change in one object may affect the other object. For example, a "Car" object may depend on a "Fuel" object to function. “uses”
- Aggregation: Aggregation represents a whole-part relationship between objects, where one object is composed of or contains other objects. It is a specialised form of association where the objects have a "has-a" relationship. For example, a "University" object can be composed of multiple "Department" objects. “a part of”
- Inheritance: Inheritance is a mechanism where an object or class acquires the properties and behaviours of another class through inheritance. It represents an "is-a relationship”, where a subclass inherits the attributes and methods of a superclass. It allows for code reuse, promotes modularity, and supports the concept of polymorphism.
- Composition: A special type of aggregation where parts are destroyed when the whole is destroyed. “made of”
D.1.7 Outline the need to reduce dependencies between objects in a given problem.
- Modularity and Maintainability: Dependencies between objects can make the system tightly coupled, where changes in one object can have a cascading effect on others. By reducing dependencies, we can enhance modularity, which allows us to break down complex systems into smaller, independent components. Modularity improves maintainability by isolating changes to specific components, making it easier to understand, modify, and update the codebase.
- Flexibility and Adaptability: Dependencies can create rigid systems that are difficult to modify or extend. When objects are highly interdependent, making changes to one object may require modifying multiple others. By reducing dependencies, we increase the flexibility and adaptability of the system. This enables easier integration of new features, addition of new components, and handling changes in requirements without impacting the entire system.
- Testability and Debugging: Dependencies can complicate the testing and debugging processes. When objects rely heavily on each other, it becomes challenging to isolate and test individual components in isolation. By reducing dependencies, we can improve testability by enabling the testing of individual objects independently. It also simplifies debugging as issues can be localized to specific components without the need to trace through a complex web of interconnected objects.
- Coupling Reduction: Dependencies and coupling are closely related. Tight coupling between objects can lead to several drawbacks, such as reduced code reusability, increased complexity, and difficulty in making changes. By reducing dependencies, we automatically reduce coupling, making the system more loosely coupled. Loose coupling promotes better encapsulation, separation of concerns, and improves the overall design of the system.
- Parallel Development: When objects have numerous dependencies, it can hinder parallel development efforts. Teams may need to wait for dependencies to be resolved before proceeding with their work, leading to delays and inefficiencies. By reducing dependencies, different teams can work on independent components simultaneously, accelerating development and enabling faster iterations.
- Code Reusability: Dependencies can limit code reusability, as objects tightly coupled to others may be difficult to extract and use in different contexts. By reducing dependencies, we enhance code reusability by creating independent and self-contained components that can be easily integrated into various systems or projects. This promotes code sharing, reduces duplication, and leads to more efficient development practices.
In summary, reducing dependencies between objects is crucial for promoting modularity, flexibility, testability, maintainability, and code reusability. It enables teams to work in parallel, simplifies debugging and testing, and provides a solid foundation for building scalable and adaptable systems.
D.1.8 Construct related objects for a given problem.
(In an exam you will be presented with a scenario (problem) and be asked to identify the objects involved and the relationships between them. You may be asked to represent this in a UML diagram as described above.)
D.1.9 Explain the need for different data types to represent data items.
Primitive Data Type
- Integer: Integer data types are used to represent whole numbers without fractional parts. They are used for counting, indexing, and representing numerical quantities. 32-bits (4 bytes) used to store the information.
- Real: Real or floating-point data types are used to represent numbers with fractional values. They are used for calculations involving decimal numbers or when precision is required. Computers actually represent approximations of real numbers with a trade-off between range and precision. The double data type has more precision and can represent larger numbers than the float. ‘Float’ uses 32-bits and ‘double’ uses 64-bits. The double is the default type in java but ‘float’ should be used for arrays of real numbers in order to save storage space.
- String: String data types are used to represent sequences of characters. They are used for storing and manipulating text or character-based data. Each character is stored in a byte of memory.
- Boolean: Boolean data types represent logical values, typically either true or false. They are used for making decisions and controlling program flow based on conditions. It occupies 1 bit of data in memory.
We need different data types because we have different sorts of data. All data must be converted into binary in order for it to be stored and processed by the computer. Each data types ‘tells’ the computer how to deal with the data it is given and how much memory space the data will use. By choosing the correct data type we can control how much memory our program will use.
D.1.10 Describe how data items can be passed to and from actions as parameters.
Parameters enable the passing of different values to a method, allowing the same method to be used with different inputs. They help in making methods more general and adaptable to different scenarios.
When parameters are passed by value, a copy of the actual data is passed to the method. Any modifications made to the parameter within the method do not affect the original data outside the method. This is the case for primitive data types and immutable objects.
When parameters are passed by reference, a reference or memory address of the actual data is passed to the method. Any modifications made to the parameter within the method directly affect the original data outside the method. This is the case for objects or mutable data types.
Procedure, Routine, Sub-procedure, Sub-routine
- Procedure: A procedure is a named block of code that performs a specific task or a series of tasks. It is a self-contained unit that can be called from other parts of the program. Procedures can have parameters, which are variables or values passed to the procedure to provide input or information necessary for its execution. Parameters allow data to be passed into the procedure, enabling it to operate on different inputs.
- Routine: A routine is a generic term that encompasses procedures, functions, methods, and subroutines. It refers to a block of code that performs a specific task. Like procedures, routines can have parameters to accept input values. Routines can also return values as output, depending on the programming language.
- Sub-procedure: A sub-procedure is a procedure that is called within another procedure or routine. It helps modularize code by breaking down complex tasks into smaller, manageable parts. Sub-procedures can have their own parameters, which may be independent of or related to the parameters of the calling procedure.
- Sub-routine: Sub-routine is another term used to describe a self-contained block of code that performs a specific task. It is similar to a procedure or routine and can have parameters for accepting input values. Sub-routine refers to a section of code that can be invoked from different parts of the program to perform a specific task.
Leave a Reply